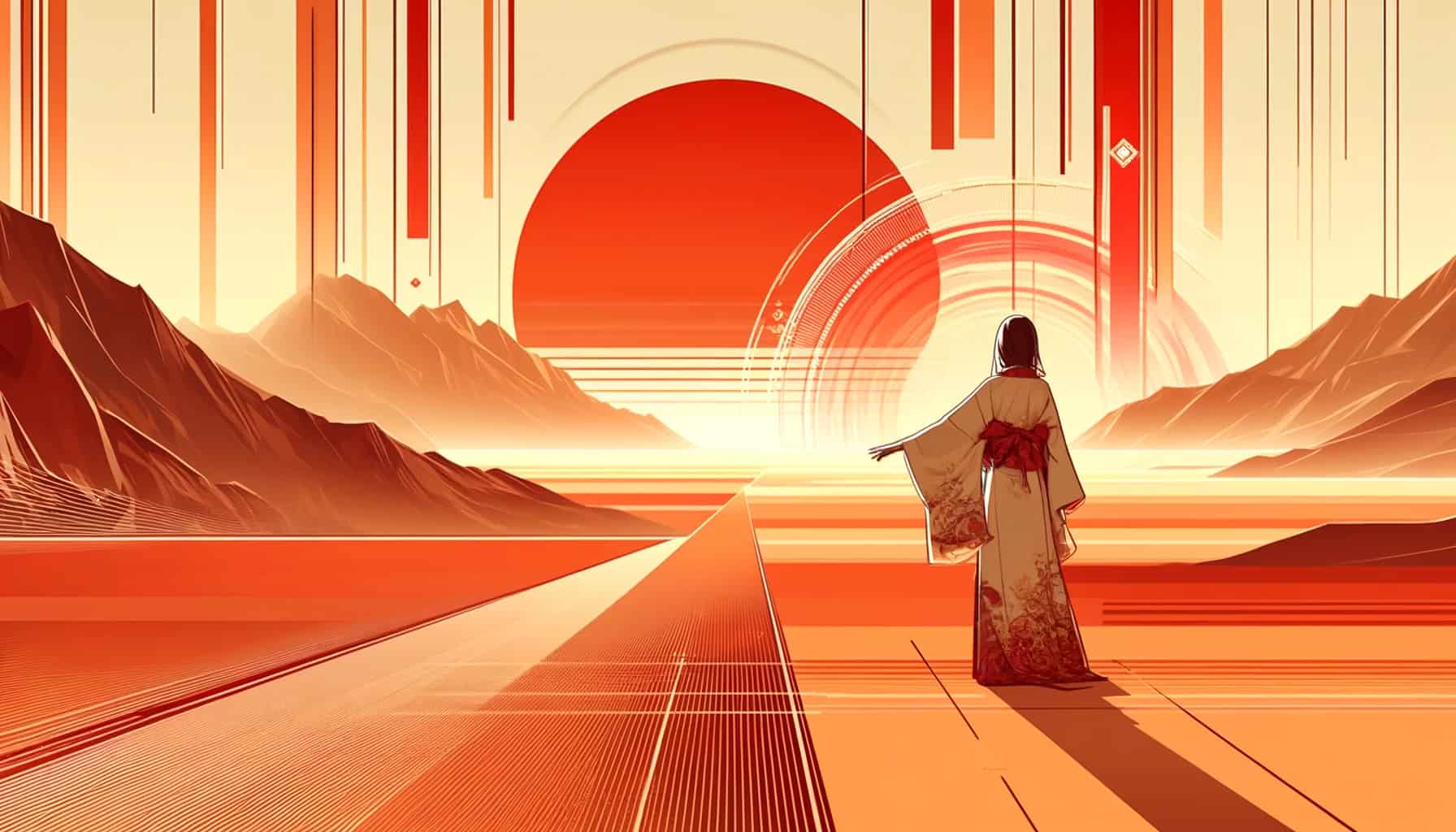
What is “pyenv”?
pyenv is a popular tool used to manage multiple versions of Python on a single machine. It allows you to easily switch between different Python versions and manage project-specific Python environments. This can be particularly useful for developers who need to test their code across different Python versions or maintain projects that rely on different Python interpreters.
Key Features of pyenv
- Python Version Management:
- Install Multiple Versions: You can install multiple versions of Python, including CPython, PyPy, Anaconda, Miniconda, Stackless, and more.
- Switch Between Versions: Easily switch between different Python versions using simple commands.
- Set Global and Local Versions: Define global and directory-specific Python versions, allowing for project-specific settings.
- Isolation:
- Environment Isolation: Ensures that projects are isolated and use the specified version of Python, preventing conflicts between dependencies.
- Integration with Virtual Environments:
pyenv-virtualenv
Plugin: Provides additional functionality to manage virtual environments, enhancing the isolation and management of dependencies.
- Compatibility:
- Cross-Platform: Works on various operating systems, including Unix-like systems (Linux, macOS) and Windows (with some additional setup).
Install pyenv
Check the Ubuntu Version
apt update
# If lsb_release is not installed, you can install it using:
apt install lsb-release
# This command provides detailed information about the Ubuntu version.
lsb_release -a
Install Dependencies
pyenv
requires some dependencies to be installed first. Open your terminal and run the following commands to install the necessary packages:
sudo apt-get update
sudo apt-get install -y make build-essential libssl-dev zlib1g-dev libbz2-dev libreadline-dev libsqlite3-dev wget curl llvm libncurses5-dev libncursesw5-dev xz-utils tk-dev libffi-dev liblzma-dev python3-openssl git
Install pyenv
Run the following command to clone the pyenv
repository from GitHub into your home directory: This script installs pyenv
, pyenv-virtualenv
, and some other useful plugins automatically.
curl https://pyenv.run | bash
Check Current Shell:
echo $SHELL
Install “vim”
sudo apt install vim
Add pyenv to your shell startup file:
You need to add the following lines to your shell startup file (~/.bashrc
, ~/.zshrc
, etc.) to configure the environment for pyenv
. This allows pyenv
to be available every time you open a new terminal session.
# If you are using bash:
echo 'export PATH="$HOME/.pyenv/bin:$PATH"' >> ~/.bashrc
echo 'eval "$(pyenv init --path)"' >> ~/.bashrc
echo 'eval "$(pyenv init -)"' >> ~/.bashrc
echo 'eval "$(pyenv virtualenv-init -)"' >> ~/.bashrc
# If you are using zsh:
echo 'export PATH="$HOME/.pyenv/bin:$PATH"' >> ~/.zshrc
echo 'eval "$(pyenv init --path)"' >> ~/.zshrc
echo 'eval "$(pyenv init -)"' >> ~/.zshrc
echo 'eval "$(pyenv virtualenv-init -)"' >> ~/.zshrc
Apply the Changes
To apply the changes made to your shell startup file, you need to source the file. This will update your current session with the new environment variables and configuration.
# For bash:
source ~/.bashrc
# For zsh:
source ~/.zshrc
Verify the Installation
To verify that pyenv
has been installed correctly, you can run the following command:
pyenv versions
This should display a list of Python versions managed by pyenv
, although initially it will be empty as you haven’t installed any Python versions yet.
Install a Python Version
# You can now use pyenv to install any Python version. For example, to install Python 3.9.7, run:
pyenv install 3.9.7
# To set this version as the default version globally, run:
pyenv global 3.9.7
# To verify that the correct Python version is being used, you can run:
python --version
Uninstall a Python Version
pyenv uninstall <version>
List Installed Python Versions
pyenv versions
Set Global Python Version
Sets the global Python version, which is used by default in all shell sessions.
pyenv global <version>
To remove the global Python version setting in pyenv, you need to unset the global version. pyenv doesn’t provide a direct command to “remove” the global version, but you can change it or clear it by setting it to system, which tells pyenv to use the system’s default Python version.
pyenv global system
- System Python Version: Setting the global version to system tells
pyenv
to use the system’s default Python interpreter, which is typically installed at a standard location like/usr/bin/python
. - Global vs Local: Remember, this change only affects the global setting. If you have local (.python-version files in directories) or shell-specific versions set, those will still take precedence when you’re in those directories or sessions.
Check Current Global Python Version
pyenv global
Set Local Python Version
Sets the local Python version for the current directory. This is useful for projects that require a specific Python version.
pyenv local <version>
When you use the command pyenv local , pyenv sets the specified Python version as the local version for the current directory. This means that whenever you are in that directory (or any subdirectory), the specified Python version will be used by default. Here’s a detailed explanation of what happens and how to use it:
What Happens
- Creates a .python-version File:
- pyenv writes the specified version to a file named .python-version in the current directory.
- The content of the .python-version file is simply the version number you specified.
- Directory-Specific Python Version:
- Whenever you or any script runs a Python command in that directory (or any subdirectory), pyenv will use the version specified in the .python-version file.
- This allows you to have different projects using different Python versions on the same system without conflicts.
- Overrides Global and Shell Versions:
- The local version specified with pyenv local overrides the global version set with pyenv global and the shell version set with pyenv shell for the current directory.
Impact of Using pyenv local
- Isolation: This ensures that the specific project uses the specified Python version, which helps in maintaining compatibility and avoiding conflicts with other projects that might require different versions.
- Portability: When you share your project with others, the .python-version file can be included in version control, ensuring that everyone uses the same Python version.
- Convenience: Automatically using the correct Python version for your project without needing to manually switch versions every time you switch projects.
Remove local Python environment
To remove a local Python environment set by pyenv local, you essentially need to delete the .python-version file from the directory. This file is what pyenv uses to determine the local Python version for that directory. Here are the steps to do this:
rm .python-version
Set Shell Python Version
Sets the Python version for the current shell session only.
pyenv shell <version>
Rehash
Rebuilds the pyenv shims. This is necessary after installing new Python versions or packages that include binaries.
pyenv rehash
List All Available Python Versions:
# To list all Python versions available for installation with pyenv, use the following command:
pyenv install --list
This command will display a list of all Python versions that can be installed via pyenv
. The list will include a variety of versions, such as:
- Official releases of CPython (e.g., 3.9.7, 3.8.10)
- Anaconda distributions
- PyPy versions
- Stackless versions
- Other distributions and versions.
Filtering Versions: You can use grep to filter the list if you are looking for a specific version or range of versions. For example, to find all 3.9.x versions, you can use:
pyenv install --list | grep " 3.9"
Virtual Environment
Create a Virtual Environment
Creates a new virtual environment using a specific Python version (requires pyenv-virtualenv plugin).
pyenv virtualenv <version> <env_name>
Looking in links: /tmp/tmpqw0i7tdj
Requirement already satisfied: setuptools in /home/ubuntu/.pyenv/versions/3.9.7/envs/myenv/lib/python3.9/site-packages (57.4.0)
Requirement already satisfied: pip in /home/ubuntu/.pyenv/versions/3.9.7/envs/myenv/lib/python3.9/site-packages (21.2.3)
Activate a Virtual Environment
Activates a specific virtual environment (requires pyenv-virtualenv plugin).
pyenv activate <env_name>
Deactivate the Current Virtual Environment
Deactivates the currently active virtual environment (requires pyenv-virtualenv plugin).
pyenv deactivate
List All Virtual Environments
Lists all virtual environments managed by pyenv (requires pyenv-virtualenv plugin).
pyenv virtualenvs
Delete a Virtual Environment
Deletes a specific virtual environment (requires pyenv-virtualenv plugin).
pyenv uninstall <env_name>
How to upgrade “pyenv”
Ubuntu
# The default installation directory for pyenv is ~/.pyenv. Change directory to this location:
cd ~/.pyenv
# Pull the latest changes from the pyenv repository:
git pull
# Restart Your Shell After updating the pyenv repository, you need to restart your shell to ensure
# that the updated version is loaded. You can do this by either closing and reopening your terminal
# or sourcing your shell configuration file.
source ~/.bashrc
Mac
# Update Homebrew/Linuxbrew:
brew update
# Upgrade pyenv
brew upgrade pyenv
# Sourcing the shell configuration file reloads the shell environment without having to close and reopen Terminal.
source ~/.bash_profile
A concise comparison between “pyenv” and Native “venv”
Overview
pyenv and pyenv-virtualenv
- Purpose: Manage multiple Python versions and their associated virtual environments.
- Key Feature: Allows seamless switching between different Python versions and virtual environments.
Native venv
- Purpose: Create lightweight, isolated Python environments using the standard library.
- Key Feature: Simple, out-of-the-box solution for environment isolation.
Multiple Python Version Management
pyenv and pyenv-virtualenv
- Pros
- Allows you to install and switch between multiple Python versions effortlessly.
- Great for projects requiring different Python versions.
- Ensures that each project can have its own isolated Python version, reducing conflicts.
- Cons
- Managing multiple versions can introduce additional complexity.
- Requires more disk space for multiple Python installations.
Native venv
- Pros
- Uses the system Python version, ensuring consistency across environments.
- Simple and straightforward for projects that do not require multiple Python versions.
- Cons
- Does not support managing multiple Python versions.
- If you need a different Python version, you must manually install it outside of venv.
Ease of Use
pyenv and pyenv-virtualenv
- Pros:
- Commands like
pyenv global
,pyenv local
, andpyenv shell
make it easy to manage Python versions. pyenv virtualenv
provides convenient commands for creating and managing virtual environments.
- Commands like
- Cons:
- Slightly steeper learning curve due to more commands and options.
- New users may find it more complex compared to the simplicity of
venv
.
Native venv
- Pros:
- Very easy to create and manage virtual environments with minimal commands.
- Ideal for beginners due to its simplicity.
- Cons:
- Less powerful in terms of managing multiple environments and Python versions.
- Limited feature set compared to
pyenv
.
Environment Isolation
pyenv and pyenv-virtualenv
- Pros:
- Provides strong isolation by linking each virtual environment to a specific Python version.
- Ensures that dependencies and Python versions are completely isolated.
- Cons:
- Managing different environments can become complex if not organized properly.
- Requires understanding of both
pyenv
andpyenv-virtualenv
commands.
Native venv
- Pros:
- Provides effective isolation of dependencies within the virtual environment.
- Simple to understand and use for isolating project dependencies.
- Cons:
- Limited to the installed system Python version, which can lead to conflicts if multiple versions are needed.
Portability and Reproducibility
pyenv and pyenv-virtualenv
- Pros:
- Ensures that the exact Python version and environment can be reproduced on different machines.
.python-version
files can be included in version control to specify the Python version for the project.
- Cons:
- Requires installation of
pyenv
on all systems where the project will be run. - Slightly more complex setup on new machines.
- Requires installation of
Native venv
- Pros:
- Easy to recreate environments using
requirements.txt
andpython3 -m venv
. - No need for additional tools beyond Python itself.
- Easy to recreate environments using
- Cons:
- Relies on the system Python version, which must be consistent across all environments.
- Can be less straightforward to ensure exact reproducibility if different Python versions are needed.
Summary
pyenv and pyenv-virtualenv
Pros:
- Manages multiple Python versions easily.
- Provides strong environment isolation.
- Ensures reproducibility across different machines.
- Flexible and powerful for advanced use cases.
Cons:
- More complex initial setup.
- Requires additional disk space for multiple Python installations.
- Slightly steeper learning curve.
Use pyenv with pyenv-virtualenv if:
- You need to manage multiple Python versions across different projects.
- You require advanced features and flexibility in environment management.
- Ensuring exact reproducibility and isolation across different machines is crucial.
Native venv
Pros:
- Simple and straightforward to use.
- No additional installation required beyond Python.
- Effective for single Python version projects.
- Ideal for beginners and simple use cases.
Cons:
- Limited to managing the installed system Python version.
- Less powerful for managing multiple environments and versions.
- Less flexibility in ensuring exact reproducibility across different setups.
Use native venv if:
- You only need to manage virtual environments for a single Python version.
- Simplicity and minimal setup are important.
- You are working on smaller projects or scripts that do not require complex environment management.